React + FastApi Stack
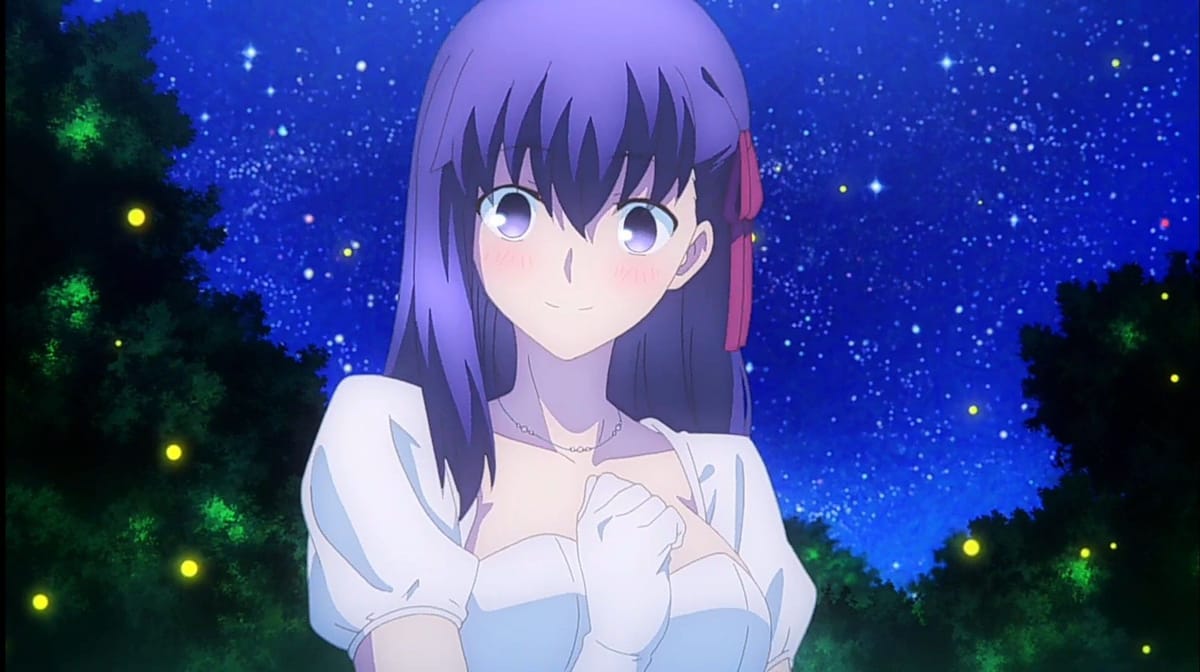
I will write about how to initailize a project using React as front-end and FastApi (python based) for back-end.
Under the project folder, firstly we create a Server
folder and initialize our back-end.
python3.10 -m venv venv
In console, type in the above command line to create a virtual environment for python.
.\venv\Scripts\Activate // in Windows
source venv/bin/activate // in Unix
Use the above line to activate the environment we just created.
set PYTHONPATH=%cd% // in Windows
export PYTHONPATH=$PWD // Unix
Set the environment variable.
Now there should be a folder called venv
under the Server
folder.
Remember, we should not track and upload venv
folder to github, since it containss system-related info and large size packages.
Outside venv
folder, and under the Server
folder, we create a file named main.py
, and a folder called app
.
└── Server
├── main.py
└── app
├── __init__.py
└── api.py
|__venv
How the stucture look like
In file main.py
:
import uvicorn
if __name__ == "__main__":
uvicorn.run("app.api:app", host="0.0.0.0", port=8000, reload=True)
//we run the back-end at port:8000
In file api.py
:
from fastapi import FastAPI
from fastapi.middleware.cors import CORSMiddleware
app = FastAPI()
origins = [
"http://localhost:3000",
"localhost:3000"
]
// we run front-end at port:3000
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"]
)
@app.get("/", tags=["root"])
async def read_root() -> dict:
return {"message": "Welcome to your back-end."}
The middleware here is FastApi default one: CORSMiddleware. We use it to handle various requests from the front-end.
Now if we visit http://localhost:8000 in local browser, we can see the message: "Welcome to your back-end."
Then, under the project folder, we initialize our React front-end.
In the console type:
npm create vite
In prompt, name the vite project as Client
, choose framework as React
, and choose TypeScript
.
Then, follow the instuction poped out:
cd Client // enter the folder we just created
npm i // install packages
npm run dev // run the front-end
Now, we can see the default count vite application on the browser.
Can see the ui page for test endpoints.
To activate python env:
.\venv\Scripts\activate
After enter the enviroment, to start the server:
uvicorn app:app --reload
To connect front-end and back-end, we need to create some endpoints in back-end, and in front-end we use axios or fetch to call those endpoints and exchange input and output.
connect to db