Learning on Backend
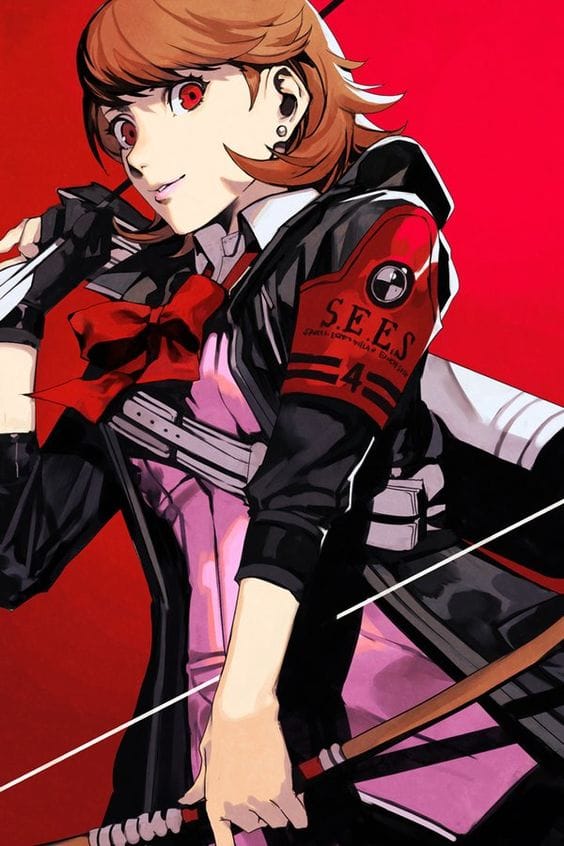
Here is the tutorial given by TA to learn how to using REST API.
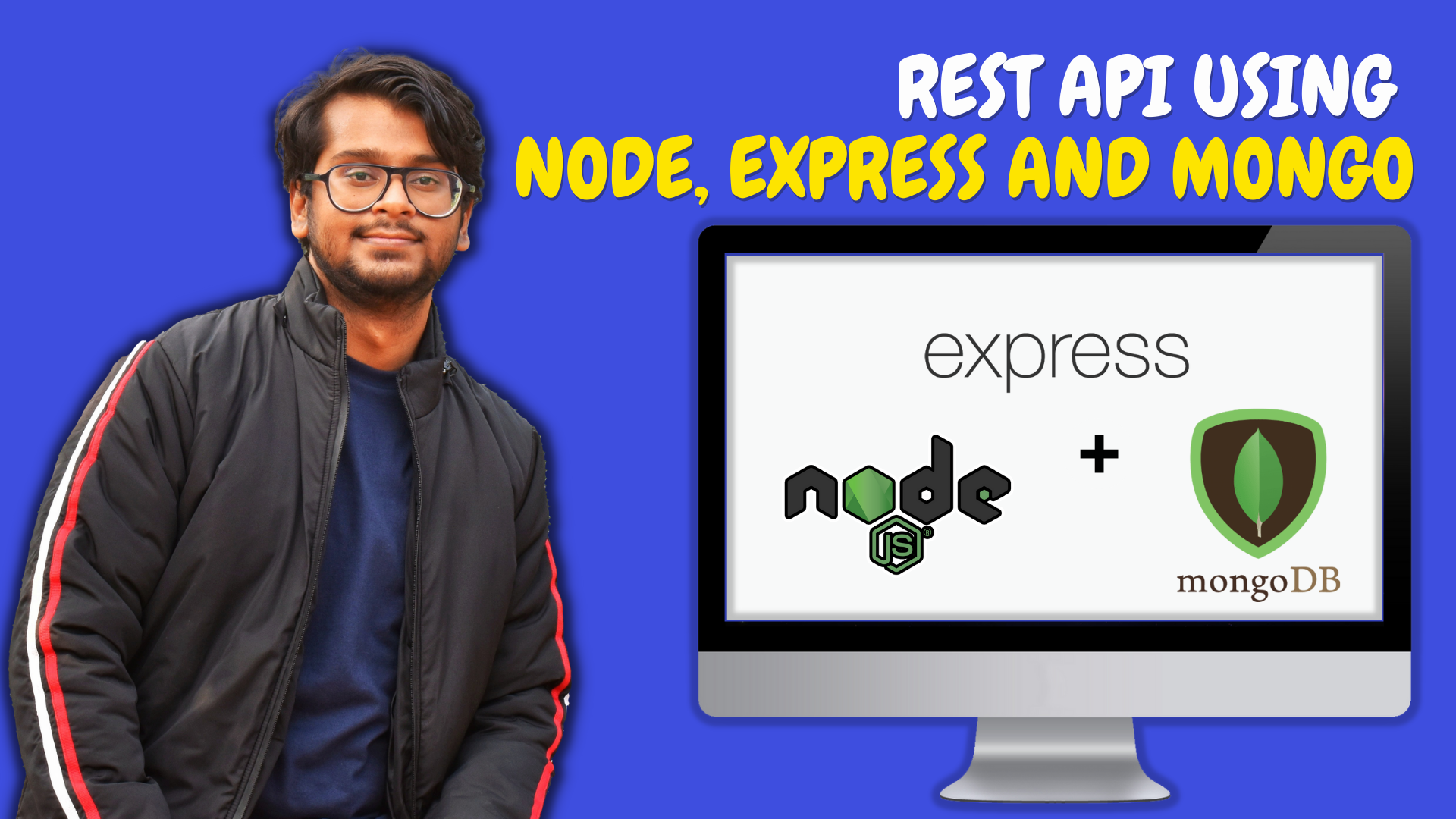
As I followed the steps, there are a few issues.
Issue 1:
yukinolov@DESKTOP-SJEKEVV:~/test_bankend$ npm start
> test_bankend@1.0.0 start
> nodemon index.js
'\\wsl.localhost\Ubuntu\home\yukinolov\test_bankend'
CMD.EXE was started with the above path as the current directory.
UNC paths are not supported. Defaulting to Windows directory.
'nodemon' is not recognized as an internal or external command,
operable program or batch file.
This error message basically means that when I try to run 'npm start', the system defauly call the path from windows directory instead of calling it in WSL. As I learned later, I installed 'npm' inside windows and when I call it, it calls from windows.
Solution:
In WSL, open the configuration file of it.
sudo nano /etc/wsl.conf
Then, add the following code into it.
[interop]
appendWindowsPath = false
This code forces us to call the command from WSL instead of windows.
Restart the WSL.
Use following cmd to check update and download nodejs and npm.
sudo apt update
sudo apt install nodejs npm
Issue 2:
yukinolov@DESKTOP-SJEKEVV:~/test_bankend$ npm start
> test_bankend@1.0.0 start
> nodemon index.js
sh: 1: nodemon: Permission denied
Solution:
Need to download nodemon in global.
sudo npm install -g nodemon
The following cmd can be used to check is nodemon is installed.
npm list -g nodemon
Issue 3:
[nodemon] starting `node index.js`
/home/yukinolov/test_bankend/node_modules/mongodb/lib/admin.js:62
session: options?.session,
^
SyntaxError: Unexpected token '.'
at wrapSafe (internal/modules/cjs/loader.js:915:16)
at Module._compile (internal/modules/cjs/loader.js:963:27)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:1027:10)
at Module.load (internal/modules/cjs/loader.js:863:32)
at Function.Module._load (internal/modules/cjs/loader.js:708:14)
at Module.require (internal/modules/cjs/loader.js:887:19)
at require (internal/modules/cjs/helpers.js:74:18)
at Object.<anonymous> (/home/yukinolov/test_bankend/node_modules/mongodb/lib/index.js:6:17)
at Module._compile (internal/modules/cjs/loader.js:999:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:1027:10)
[nodemon] app crashed - waiting for file changes before starting...
-----------------------------OR-----------------------------------------------
yukinolov@DESKTOP-SJEKEVV:~/test_bankend$ npm start
> test_bankend@1.0.0 start /home/yukinolov/test_bankend
> nodemon index.js
[nodemon] 3.1.0
[nodemon] to restart at any time, enter `rs`
[nodemon] watching path(s): *.*
[nodemon] watching extensions: js,mjs,cjs,json
[nodemon] starting `node index.js`
/home/yukinolov/test_bankend/node_modules/bson/lib/bson.cjs:593
inspect ??= defaultInspect;
^^^
SyntaxError: Unexpected token '??='
at wrapSafe (internal/modules/cjs/loader.js:1029:16)
at Module._compile (internal/modules/cjs/loader.js:1078:27)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:1143:10)
at Module.load (internal/modules/cjs/loader.js:979:32)
at Function.Module._load (internal/modules/cjs/loader.js:819:12)
at Module.require (internal/modules/cjs/loader.js:1003:19)
at require (internal/modules/cjs/helpers.js:107:18)
at Object.<anonymous> (/home/yukinolov/test_bankend/node_modules/mongodb/lib/bson.js:4:14)
at Module._compile (internal/modules/cjs/loader.js:1114:14)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:1143:10)
[nodemon] app crashed - waiting for file changes before starting...
Those error messages mean that the Node.js version is not compatible.
Solution:
Install a Node Version Manager (nvm).
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.1/install.sh | bash
Intall Node.js 15.0.0 or later.
nvm install 15
nvm use 15
Then 'npm start' should work.
Issue 4:
After successfully running 'npm start' , if you want to end it, can use following cmd.
cmd to check running process on Port 3000:
sudo lsof -i :3000
cmd to terminate the process via its PID listed by last cmd:
kill -9 [PID]
3/1/2024
Will continue setting up tmr.
The Full Process:
- initializes a new Node.js project, and necessary files to run the app will be inside.
npm init
- install a few dependencies.
npm i express mongoose nodemon dotenv
Express will be used for the middleware to create various CRUD endpoints.
Mongoose for managing data in MongoDB using various queries.
Nodemon to restart our server every time we save our file.
Dotenv to manage a .env file.
- create file index.js
const express = require('express'); // add express
const mongoose = require('mongoose'); // add mongoose
const app = express();
app.use(express.json()); // app use the func of middleware express
// and set the format into .json
app.listen(3000, () => { // server is set on Port 3000
console.log(`Server Started at ${3000}`)
})
- add script in package.json to let us start server by using npm start, nodemon will be called to restart server is there is any change in file.
"scripts": {
"start": "nodemon index.js"
},
At this point the server should work.
- in the website of mongoDB, create a project and set up a database deployment.
Choose free shared cluster, set up unsername, password, and local ip.
- copy down the connection string . ***** should be password.
mongodb+srv://nishant:********@cluster0.xduyh.mongodb.net/testDatabase
- create a file .env, and paste following string into it.
DATABASE_URL = mongodb+srv://nishant:********@cluster0.xduyh.mongodb.net/testDatabase
- in MongoDB Compass, add the string in new connection.
- connect database to our server. In index.js
require('dotenv').config(); // import .env
const mongoString = process.env.DATABASE_URL // store connection str into a var
mongoose.connect(mongoString); // connect to the database
const database = mongoose.connection
database.on('error', (error) => { // do log
console.log(error)
})
database.once('connected', () => {
console.log('Database Connected');
})
- create routes. Create a folder routes, and make a file routes.js in it.
const express = require('express');
const router = express.Router()
module.exports = router;
add code in index.js.
const routes = require('./routes/routes'); // import routes.js
app.use('/api', routes)
//app.use takes two things. One is the base endpoint, and the other is the contents of the routes. Now, all our endpoints will start from '/api'.
At this point our server should connect to database.
- write endpoints.
Each func should be one of CRUD (Create, Read, Update, Destroy)
We can use Postman to test those func.
3/2/2024